Get Query String & Path parameters in Lambda with API Gateway
In this article, we're going to discuss how to get query string and path parameters from API Gateway in AWS Lambda.
API Gateway exposes
queryParameters
and pathParameters
as properties in the event object.You can access like
event.queryParameters?.userId
or event.pathParameters?.id
.What is the problem that we're trying to solve?
When you're building Serverless API using API Gateway and Lambda, there may be scenarios where you want to access query string parameters or path parameters.
For example, you may want to fetch the user information based on the user id
parameter passed to the API. As this is a GET
request, there are primarily 2 ways to pass this information via URL - path parameter or query string parameter
In the below URL, Â user id information is passed as a path parameter 1
https://your-api-gateway-url/users/1
If you want to use a query string, you would pass the parameter after ?
as shown below
https://your-api-gateway-url/users?userId=1
Note: If you want to pass multiple values, it is better to use query string
.
https://your-api-gateway-url/users?param1=value1¶m2=value2
If you want to expose additional operations for that parameter( user id
in this case), it is better to use the path parameter as shown below.
You can use the first URL Â when want to get the user groups for the user and you can use the second URL when you want to get the metadata about the user.
https://your-api-gateway-url/users/1/groups
https://your-api-gateway-url/users/1/metadata
Infrastructure Creation
We'll be using AWS CDK
for creating all necessary AWS resources in this article. It's an open-source software development framework that lets you define cloud infrastructure. AWS CDK
supports many languages including TypeScript, Python, C#, Java, and others. You can learn more about AWS CDK from a beginner's guide here. We're going to use TypeScript in this article.
Creating REST API
We can use the below code to create REST API
const api = new RestApi(this, "queryStringPathParamApi", {
restApiName: "query-string-path-param-api",
});
Lambda function properties
We're going to use node 18
runtime for the lambda - as shown below
const nodeJsFunctionProps: NodejsFunctionProps = {
bundling: {
externalModules: [
"aws-sdk", // Use the 'aws-sdk' available in the Lambda runtime
],
},
runtime: Runtime.NODEJS_18_X,
timeout: Duration.seconds(30), // Api Gateway timeout
};
const qsPathLambdaFn = new NodejsFunction(this, "qsPathLambdaFn", {
entry: path.join(__dirname, "../lambdas", "users.ts"),
...nodeJsFunctionProps,
functionName: "qsPathLambdaFn",
});
Lambda function
In this lambda function, we're going to access the query string and path parameters from the URL. We're returning JSON with query parameters and path parameters populated from the incoming APIGateway event itself.
import { APIGatewayEvent } from "aws-lambda";
export const handler = async (event: APIGatewayEvent): Promise<any> => {
return {
statusCode: 200,
body: JSON.stringify({
queryParameters: event.queryStringParameters || {},
pathParameters: event.pathParameters || {},
}),
};
};
Integrate with API Gateway
We can integrate the lambda with API Gateway, as below.
const qsPathLambdaIntegration = new LambdaIntegration(qsPathLambdaFn);
const usersResource = api.root.addResource("users");
usersResource.addMethod("GET", qsPathLambdaIntegration);
const userResource = usersResource.addResource("{id}");
userResource.addMethod("GET", qsPathLambdaIntegration);
Please note that we've used the same lambda function for the root API resource and its child resource {id}
Deployment & Testing
You can deploy the stack using the below command
cdk deploy
Getting the query string value
If you're using query string
, you can get the value of query string parameter values from event.queryParameters?.userId
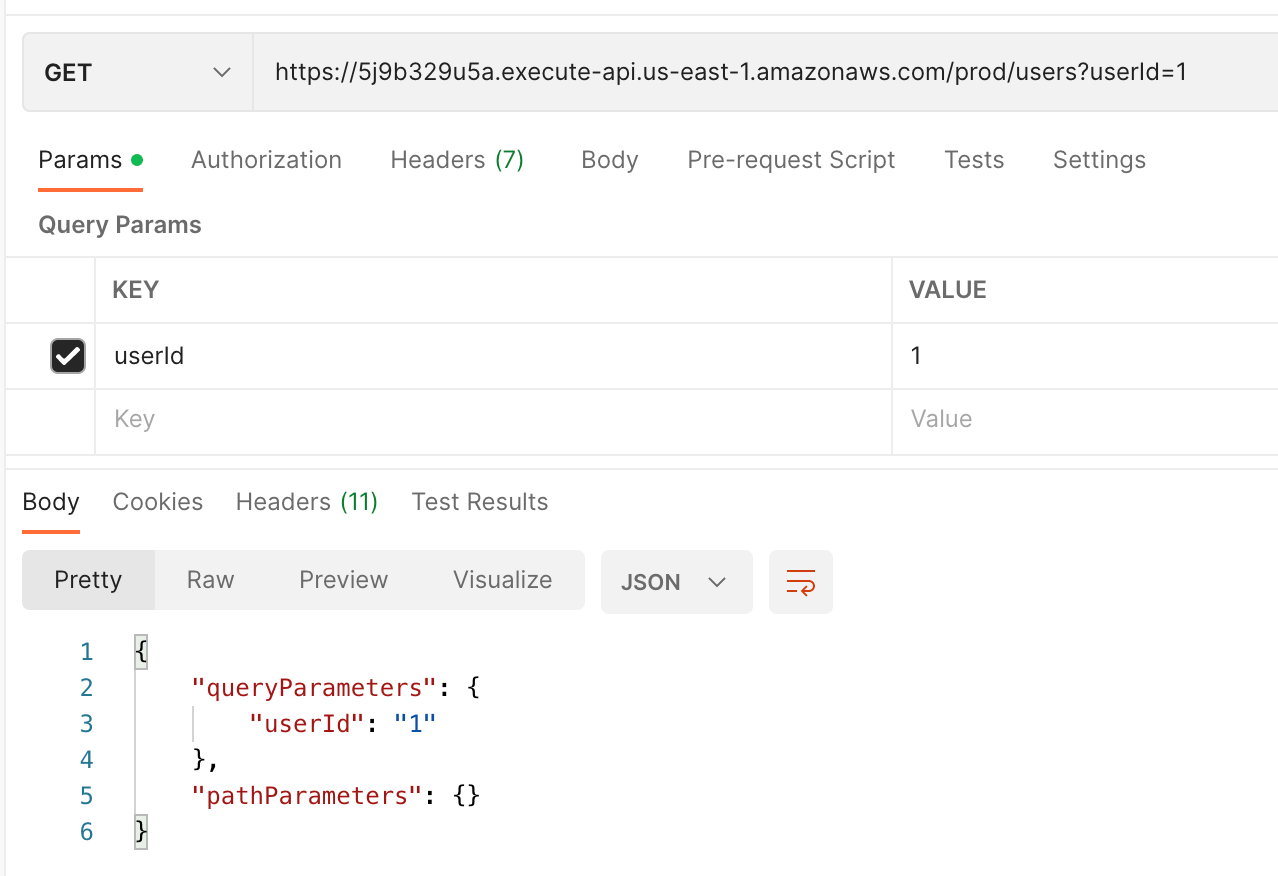
Get the path parameter values
You can access the path parameter from event.pathParameters?.id
if you've created a child resource with id
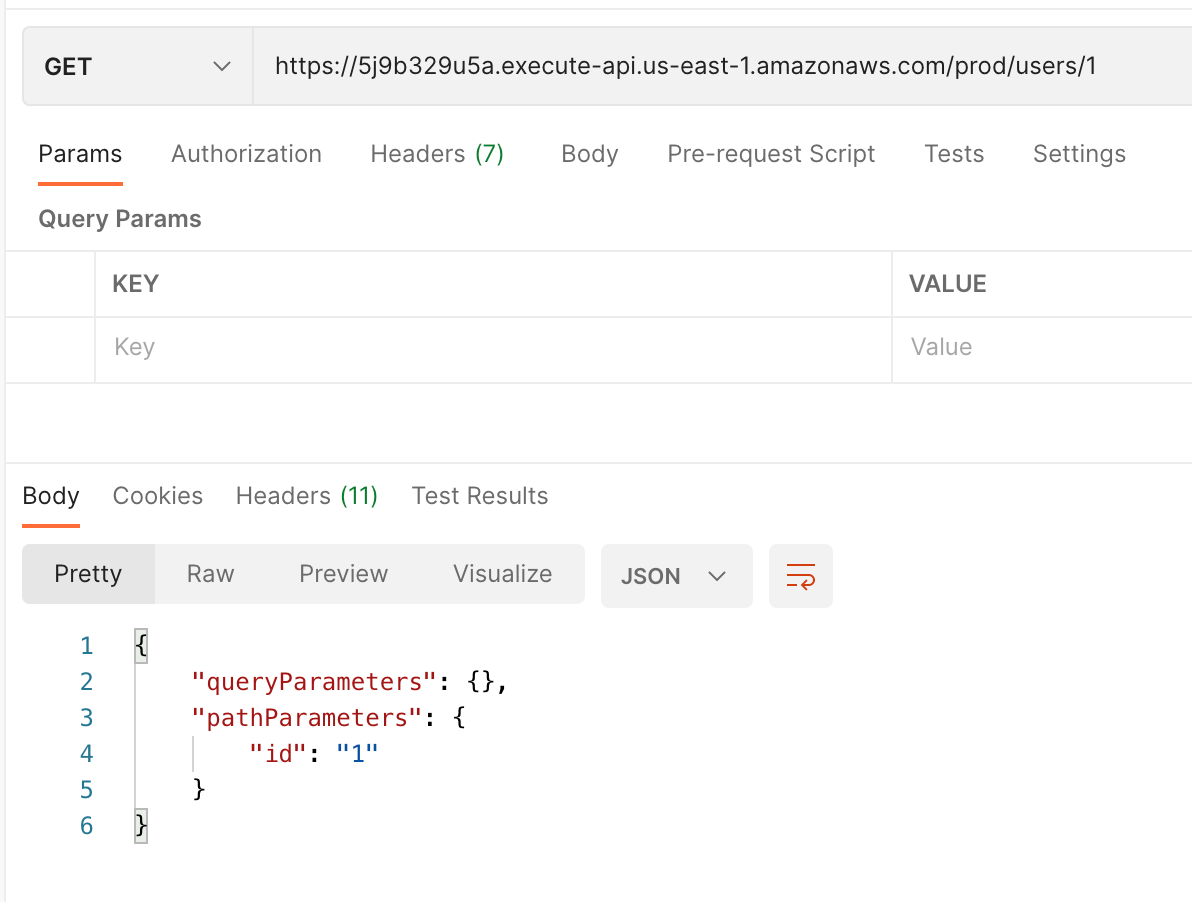
You can use these values to query the database and return the appropriate results to the user.
Please let me know your thoughts in the comments.