How to run Fastify app in AWS Lambda
In this article, we're going to discuss about how to run Fastify app in AWS Lambda.
If you're not aware of Fastify, Fastify is one of the high performant web frameworks on NodeJS.
@fastify/aws-lambda
package to bridge between AWS Lambda and Fastify applicationWhy you may want to run Fastify app in AWS Lambda?
If you have an existing Fastify application for your API, you many want to run that app in AWS Lambda because of the cost benefits and lower overhead in maintaining the infrastructure.
Infrastructure
We're going to use AWS CDK for creating the necessary infrastructure. It's an open-source software development framework that lets you define cloud infrastructure. AWS CDK supports many languages including TypeScript, Python, C#, Java, and others. You can learn more about AWS CDK from a beginner's guide here. We're going to use TypeScript in this article.
Fastify app:
We're creating a simple fastify app - as shown in below code snippet. It just has a single endpoint with 2 methods - one for GET
request and another for POST
request
import Fastify from "fastify";
const app = Fastify();
app.get("/", async (request, reply) => {
return { msg: "Hello! from Fastify!" };
});
app.post("/", async (request, reply) => {
const body = request.body;
return { msg: "Fastify received your post request!", body };
});
export default app;
Integrating Fastify app with AWS Lambda
There is a package @fastify/aws-lambda
which we need to install to integrate AWS Lambda with Fastify app.
As shown in the below code snippet, we need to wrap the Fastify app with the awsLambdaFastify
function exposed by @fastify/aws-lambda
package which would return proxy. This proxy
function acts as a bridge between Fastify app and AWS Lambda.
import awsLambdaFastify from "@fastify/aws-lambda";
import app from "./app";
const proxy = awsLambdaFastify(app);
export async function handler(event: any, context: any) {
return await proxy(event, context);
}
You just need to call the proxy
function and returns the result.
Lambda Function
We can create lambda function using CDK as shown below.
const nodeJsFunctionProps: NodejsFunctionProps = {
bundling: {
externalModules: [
"aws-sdk", // Use the 'aws-sdk' available in the Lambda runtime
],
},
runtime: Runtime.NODEJS_18_X,
timeout: Duration.seconds(30), // Api Gateway timeout
};
const fastifyFn = new NodejsFunction(this, "fastifyFn", {
entry: path.join(__dirname, "../lambdas", "fastify-lambda.ts"),
...nodeJsFunctionProps,
functionName: "fastifyFn",
});
REST API and Lambda Integration
In below code snippet, we are creating a REST api and lambda integration. Please note that we're having this lambda integration for ANY
method. It means the requests would be routed to our fastify app for all the methods such as GET
, POST
, PUT
, DELETE
etc..
const api = new RestApi(this, "fastifyApi", {
restApiName: "fastify-api",
});
const lambdaIntegraton = new LambdaIntegration(fastifyFn);
api.root.addMethod("ANY", lambdaIntegraton);
Deployment & Testing
We can deploy the stack by executing cdk deploy
command and you can test the API endpoint from Postman
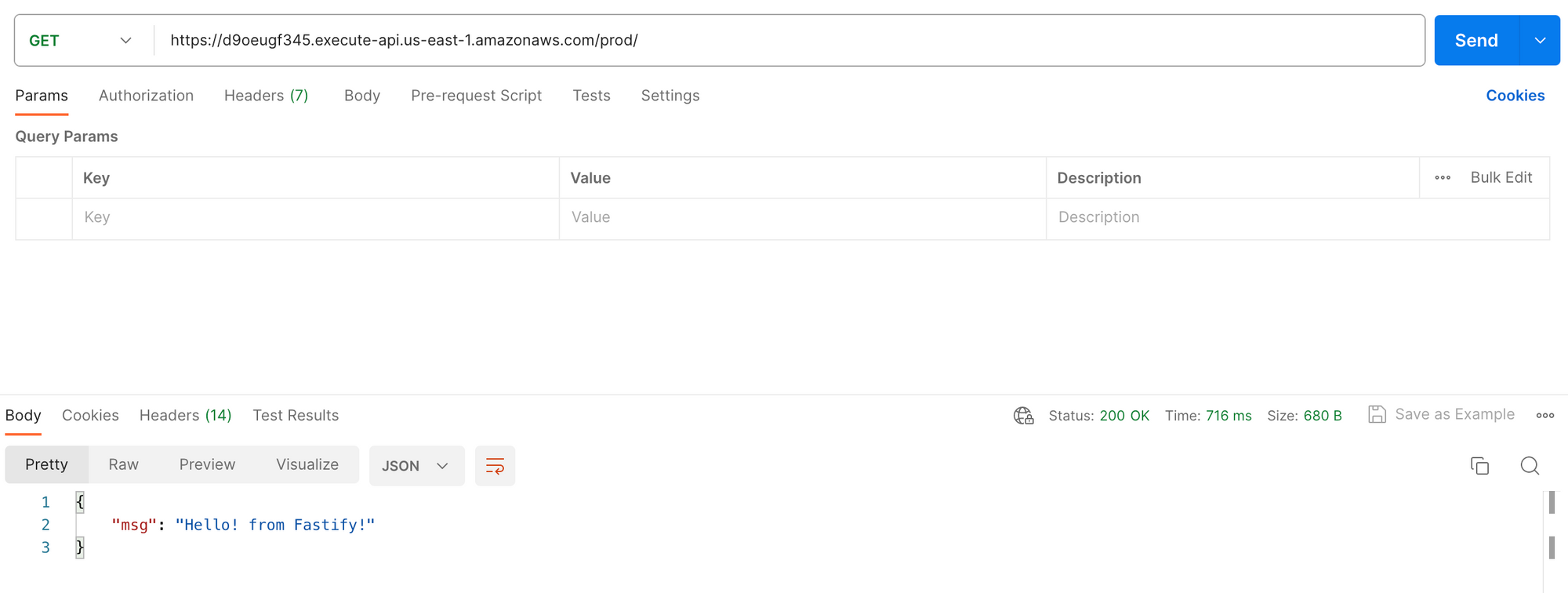
Please let me know your thoughts in the comments.